:: Tutorial :: Quick Start ::
This page quickly aquaints you with the major features of
the Forms framework. Find more information on the other tutorial pages
and details in the Reference.
Working with the FormLayout is basically a six step process:
- Find the layout
- Find the Grid
- Create the layout: specify columns and rows
- Group columns and rows
- Create and configure a builder
- Add Components
A Sample Layout
We want to implement an editor for
six fields that are separated into two groups.
General: company name and contact person;
propeller: PTI, power, radius, diameter.
Step 1: Find the Layout
Before you construct and implement a design with the Forms,
find the layout. Play around with different layouts and
evaluate how they meet your requirements.
A) uses titled borders to indicate the sections.
These prevent you from aligning components
and often bring 1 to 3 unnecessary lines.
B) uses titled separators and is cleaner.
C) uses a gap to indicate the two sections.
Gaps are perceived as separators. If section titles
are obsolete, gaps are a good choice.
D) puts related text fields into the same rows.
|
|
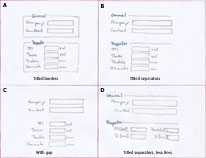
Playing With Designs
|
Step 2: Find the Grid |
We've choosen to go with design draft D).
Since FormLayout is grid-based,
we need to find the grid, i. e. the columns and rows.
We have columns for the leading labels, for the PTI and R fields,
a second label column and a second field column.
|
|
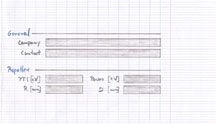
Design Draft
|
|
We add gap columns and gap rows between all component columns
and rows. Then we mark all columns and rows
and add notes for the size, and orientation.
In this tiny example, no column grows if the container grows,
otherwise we would add growing information too.
|
|
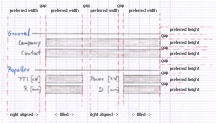
Grid, Sizes & Orientations
|
Step 3: Create the Layout: Specify Columns and Rows |
We transform the sizes and orientations into the
Forms layout specification language.
Next, we implement this layout with the Forms.
We create an instance of FormLayout
and specify the columns and rows using strings.
We abbreviate the row specs to save space.
|
|
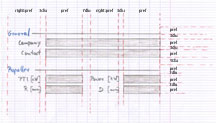
Grid Specification
|
|
FormLayout layout = new FormLayout(
"right:pref, 3dlu, pref, 7dlu, right:pref, 3dlu, pref", // columns
"p, 3dlu, p, 3dlu, p, 9dlu, p, 3dlu, p, 3dlu, p"); // rows
|
Step 4: Specify Column and Row Groups |
The label and field columns shall get the same width.
In FormLayout this is done by grouping columns;
the same can be done with rows.
To group columns, you specify an array of column indices.
Since we have two groups, we have two arrays of such indices;
these are in turn combined in an array.
|
|
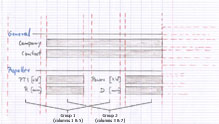
Group Specification
|
|
// Specify that columns 1 & 5 as well as 3 & 7 have equal widths.
layout.setColumnGroups(new int[][]{{1, 5}, {3, 7}});
|
Step 5: Create and Configure a Builder
In Forms you typically add components using a builder
that in turn adds them to the layout container.
Builders helps you create frequently used components,
keep track of the location for the next component
and assist you in style guide compliance.
Some builders can be configured (for example the DefaultFormBuilder).
The panel-oriented builders can set standardized borders.
PanelBuilder builder = new PanelBuilder(layout);
builder.setDefaultDialogBorder();
Step 6: Add Components
Finally we add the components. We reuse a
CellConstraints object for all cells.
// Obtain a reusable constraints object to place components in the grid.
CellConstraints cc = new CellConstraints();
// Fill the grid with components; the builder can create
// frequently used components, e.g. separators and labels.
// Add a titled separator to cell (1, 1) that spans 7 columns.
builder.addSeparator("General", cc.xyw(1, 1, 7));
builder.addLabel("Company", cc.xy (1, 3));
builder.add(companyField, cc.xyw(3, 3, 5));
builder.addLabel("Contact", cc.xy (1, 5));
builder.add(contactField, cc.xyw(3, 5, 5));
builder.addSeparator("Propeller", cc.xyw(1, 7, 7));
builder.addLabel("PTI [kW]", cc.xy (1, 9));
builder.add(ptiField, cc.xy (3, 9));
builder.addLabel("Power [kW]", cc.xy (5, 9));
builder.add(powerField, cc.xy (7, 9));
builder.addLabel("R [mm]", cc.xy (1, 11));
builder.add(radiusField, cc.xy (3, 11));
builder.addLabel("D [mm]", cc.xy (5, 11));
builder.add(diameterField, cc.xy (7, 11));
// The builder holds the layout container that we now return.
return builder.getPanel();
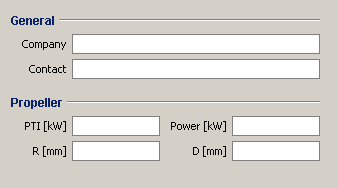
The Finished Panel
|